What does NULL mean?
Adding meaning to nullable objects significantly increases the complexity of your codebase.
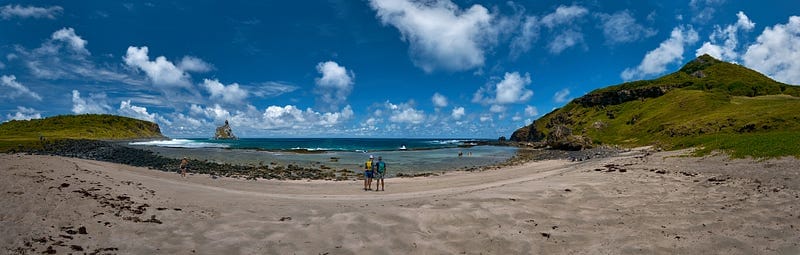
The concept of a null something exists in basically every programming language. It’s part of the architecture of a computer. You might have a memory pointer that has no address or points to an invalid memory address. This goes all the way back to 1965, during the design of ALGOL.
Fast forward to modern software development and you can have an input form with optional fields. Those will eventually be mapped to nullable properties, that might make their way to nullable columns on a table.
Everything’s in order until something happens: a null property gains business meaning.
Typically the meaning of a null property relies on previous knowledge. The team involved in that decision might carry that experience, but not newcomers.
The null condition of a property isn't clearly named, which deepens the complexity of understanding the hidden meaning.
Let’s suppose you have a user object and the Product team tells you they need to store the user’s work shift, to control login access.
However, they also mention that some users will have unrestricted login time.
Many developers would quickly solve this as: add a nullable shift property to user, and if it’s null, it means they have unrestricted access.
This works, until six months later a new developer comes to team and finds a line of code such as:
return user.admin || user.shift === null;
The logic behind this test is not instantly clear.
Instead, it would be obvious if it had been tackled with an extra property:
return user.admin || user.unrestrictedLogin;
Another classic example of null with value is nullable boolean properties. False and true have meanings, but then null also means something.
status = user.active === null ? 'Deleted' : user.active? 'Active' : 'Inactive';
These are strong candidates to enums. These little structures often go unnoticed, however they can immensely help your codebase by being very explicit on the possible values and their names:
enum AccountStatus {
ACTIVE: 'Active';
INACTIVE: 'Inactive';
DELETED: 'Deleted';
}
status = user.accountStatus.toString();
This is such a big issue that some languages are void safe, such as Kotlin and Swift.
In future posts, I’ll keep exploring one of the traits of a great codebase: obviousness.
Make your code obvious. Your company, team and your future self will thank you.